Checkout the Project
- Download or clone the demo project on on Github
- If you don't want to use bower, download the latest distribution from
web-components.fact-finder.de and unzip
the content in the demo project root directory
Depending on whether you are using the NodeJs/Bower way you need to continue with the following
steps:
NodeJs/Bower
- Run
node start.js demo-folder-name
like stated on the project
page
Without NodeJS/Bower
- Copy the project directoy into the web server of your trust. Open a html file.
Take a look at one of our many example implementation
As a starting point you can take a look at our demonstration
overview. Each demo contains a use-case
based explanation on how to achieve certain functionality and the Api-Reference of all necessary
elements.
If want to find a specific element use the element elements
overview
Styling Elements
Style Encapsulated HTML
Web Components can by styled via CSS like regular HTML. Some elements have an internal HTML structure.
Because of the style encapsulation of Shadow DOM those elements can no longer be reached as usual.
Because the /deep/
and ::shadow style rules are deprecated, custom properties and
mixins have been introduced in order to pass on styles accross the borders of Shadow DOM. You can
find all mixins and custom properties in the documentation or the html files of the individual elements.
Example for the internal HTML structure of the ff-paging-item:
In order to style the div inside this element the---paging-item-cursor-mixin
was introduced.
To overwrite the default styles the following code can be used:
It is important, that the style element possesses the property "is" with the value "custom-style".
Prevent FOUC (Flash Of Unstyled Content)
In browsers where web components are not natively supported you might encounter ugly flash of unstyled
content on page load. To prevent this we added a remove unresolved attribute behavior to all
elements
with an visual component (e.g. ff-record-list, ff-asn and so on).
Just annotate all elements which are shown immediately on page load with the [unresolved]
attribute. In Addition add the following CSS rule on top of the page before the appearance of your
custom elements:
Full example would look like:
NOTE: This behavior was released with version 1.0.8 and is therefore not supported in 1.0.0!
To check if you are already using version 1.0.8 type: factfinder.communication.version
in
your browser
console -> undefined means you are not using 1.0.8
Template Engine
Basics
All {{data-bindings}} used in FACT-Finder Web Components refer to the underlying JSON response returned by
FACT-Finder.
You can take a look at all available bindings by opening your browsers
Dev Tools (
Press F12
Key) and
navigating to the
Network tab. Filtering by
XHR makes things easier.
Now invoke the
search, two xhr
requests will appear. The second one is the one we are looking for. Click the request url, a second window
on the right will appear. Navigate to the
Preview tab to see the data as a JSON tree.
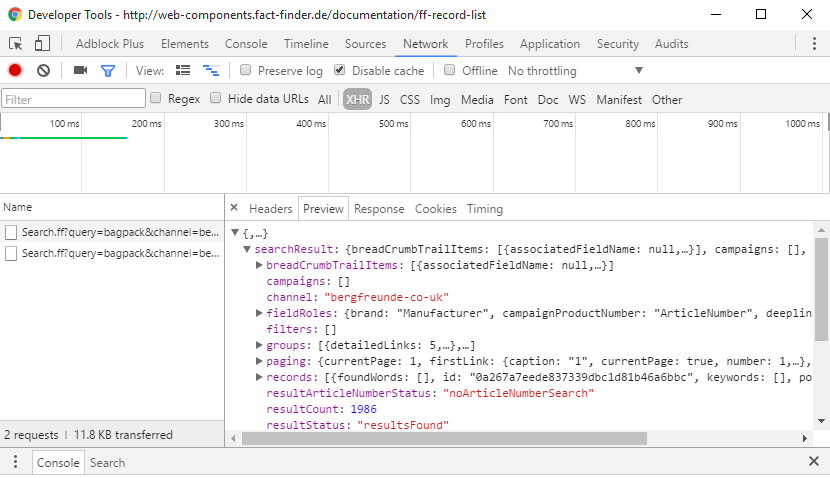
Every element with an visual component is bound to one or more of these objects. For example the ff-record
element is bound to an item in the records array.
Data Binding Example
By expanding the records array and one of it's items we can take a look at the fields returned by
FACT-Finder. In this specific example product data is stored in an record object. All fields returned in
this object are imported during the data feed process. You can configure which fields are returned in the
FACT-Finder backend.
Take a look at the following data-binding example to see how to access the data.
Underlying Engine (Mustache)
All bindings are resolved by the underlying template engine
mustache.js. For a bit more flexibility you can rely on some of it's functionality.
You could do an
if by using
this syntax. If the data is
not
formatted correctly, use the
ResultDispatcher to modify it accordignly.
FACT-Finder Web Components Demos
This is an overview of FACT-Finder Web Components Demos and documentation by Topic.
For an quick overview of each element use the Element
Overview
Basics
Navigation
More Features
Special Cases
FACT-Finder Web Components Element
This is an overview of allFACT-Finder Web Components Elements.
Basics
Navigation
More Features
Communication
By default Web Components are communicating directly with FACT-Finder. This is configured in the
ff-communication
element.
All actions and requests are performed automatically for you depending on the element was
clicked. This means for example if the user clicked a ff-asn-group-element
a filter
action is executed. FACT-Finder Web Components are basically data driven. They expect a service
which is capable of understanding the FACT-Finder API invocations and to retrieve the data like
FACT-Finder would retrieve it. See the following image:
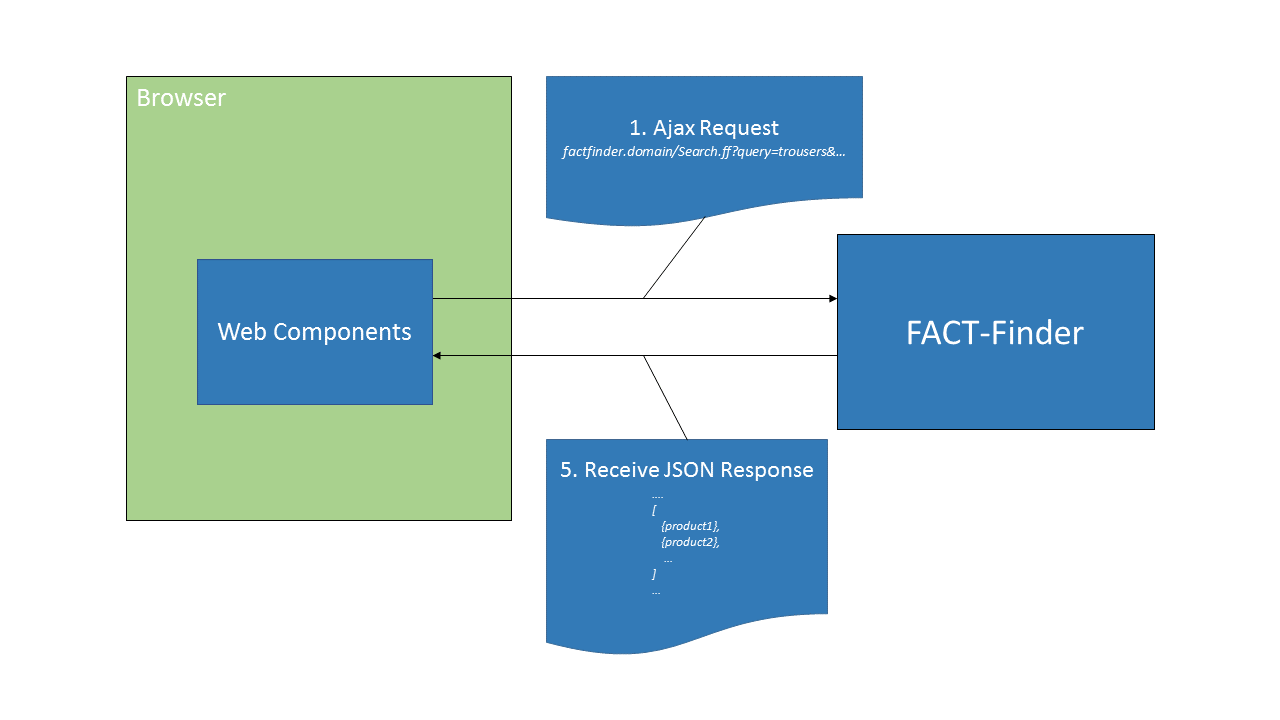
Change the Communication Flow
In some situations you don't want FACT-Finder Web Components to communicate directly with
FACT-Finder. Enriching the product data with the latest stock might be one of these use cases.
In such a case you need to take care about some simple but improtant things. You can configure our
Web Components to communicate with your shop backend by changing the [url]
attribute on
element.
Please see the following image for an rough overview of communication:
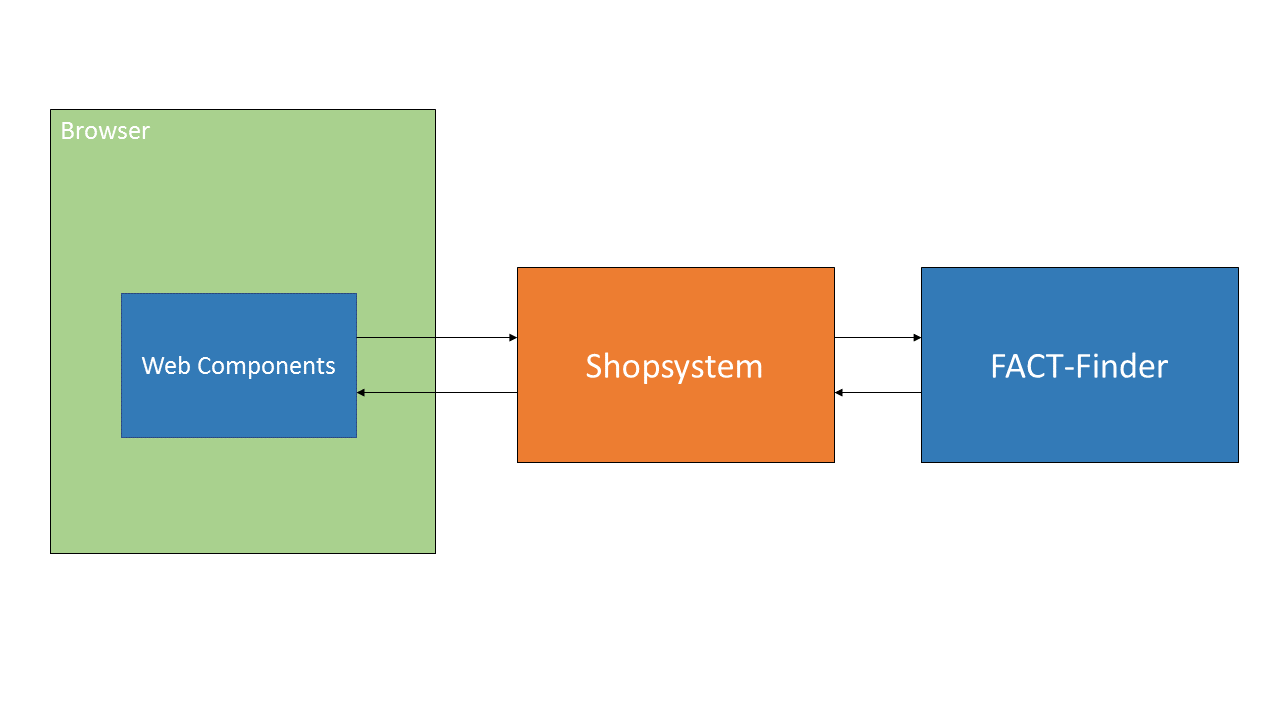
Detailed Overview of Communication
The following image illustrates the 5 steps to enrich search response with latest data from 3rd party
services. Steps marked in orange are meant to be performed by the
shop. Steps in blue are meant to be performed automatically
by Web Components.
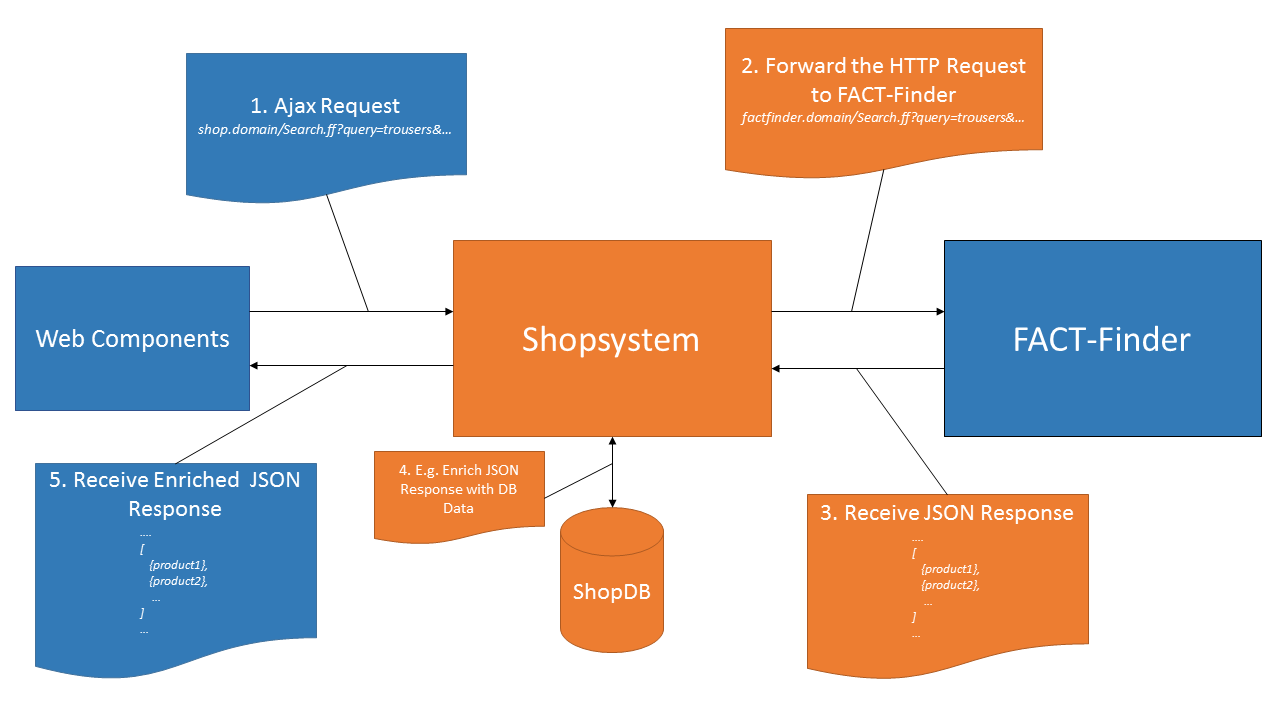
1. Ajax Request
When the user interacts with the search result page a Ajax Request is made at certain points. This
Ajax Requests needs now to be made to the shop system now. Change the url settings in ff-communication
element.
2. Forward the HTTP Request to FACT-Finder
The request made by Web Components will look something like:
http://domain.com/FACT-Finder/Search.ff?query=shoe&channel=shoeshop_en
.
The URL will always follow this pattern:
[protocol]://[domain + port]/[APP-Name]/[API-Name].ff?[HTTP-Params]
So basically all you need to do is strip everything before the API-Name away and replace it by the
original FACT-Finder URL. Taking the URLs from Step 1. one the url changes would like:
http://your.shop-endpoint.com/FACT-Finder-Endpoint/Search.ff?query=shoe&channel=shoeshop_en
http://your.factfinder.com/FACT-Finder/Search.ff?query=shoe&channel=shoeshop_en
3. Receive JSON Respone
Now you just need to make the HTTP GET request and receive the data.
4. Enrich JSON Response with additional data
Lets say you want to add latest stock to your received records. Lets consider the following
response:
The record
property is the correct place to add the product specific data. Just get the
stock data wherever you want to get it from and add it to the json response. The result would like:
5. Return Data to Web Components
You are done with manipulating the JSON response. Just send the data back and take advantage of your
enriched search response.
Web Components Core
You can subscribe to the ResultDispatcher for raw data or get a chance to play around with result
before it's dispatched. This is indeed what all data related FACT-Finder Web Components do. They
subscribe to ResultDispatcher to get notified when new data is available. If you want to trigger
your own communication events checkout the FFCommunicationEventAggregator.
Web Components Core
You can subscribe to the ResultDispatcher for raw data or get a chance to play around with result
before it's dispatched. This is indeed what all data related FACT-Finder Web Components do. They
subscribe to ResultDispatcher to get notified when new data is available. If you want to trigger
your own communication events checkout the FFCommunicationEventAggregator.
factfinder.communication.ResultDispatcher
ResultDispatcher.subscribe(topic, fn,
ctx)
Get notified when new data is available. If so the function supplied in fn
is
invoked with data according to the topic.
List of topics to subscribe for.
This is the current execution order e.g. campaigns are dispatched
before the asn is dispatched but its nor recommended to rely on this order. Use addCallback
instead.
- "result"
- "campaigns" - special
- "records"
- "query"
- "suggest"
- "bct"
- "sort"
- "ppp"
- "paging"
- "pagingItems"
- "asn"
- "singleWordSearch"
- "productDetail"
- "productCampaign" -special
- "singleWordSearch"
ResultDispatcher.unsubscribe(topic, key)
Unsubscribe during runtime for a specific topic
ResultDispatcher.addCallback(topic, fn, context)
addCallback is similar to subscribe but is guaranteed to be executed before subscribe.
ResultDispatcher.removeCallback(topic, key)
FFCommunicationEventAggregator
factfinder.communication.FFCommunicationEventAggregator
Use an event based function to query the FACT-Finder APIs. Every event needs to have a "type"
property to specify how to handle the event.
NOTE: Each property which is not not a function or an object is translated to an http request
parameter
with a few exceptions:
- "type"
- "url"
- "fail"
- "always"
- "success"
fail
, always
and success
are expected to be a function which is
executed at the appropriate time. The type
property tells the EventAggregator how to handle
the event. The url
property is reserved for internal usage.
FFCommunicationEventAggregator.addBeforeDispatchingCallback(fn):string;
FFCommunicationEventAggregator.removeBeforeDispatchingCallback(key):boolean;
Adds or removes a callback which executes before every event i.e. search, suggest,
recommendation and so on.
The callback receives an event object enriched with basic data i.e. sid
and if available a
type
property which corresponds to the event types of FFCommunicationEventAggregator.addFFEvent(event)
.
If you want to intercept a search request for example you could do:
FFCommunicationEventAggregator.addFFEvent(event)
"search" Example
"navigation" Example
"filter" Example
"bct" (breadcrumb trail) Example
"suggest" Example
"sort" Example
"ppp" (products per page) Example
"paging" (pagination) Example
"recommendation" Example
"productCampaign" Example
"shoppingCartCampaign" Example
"similarProducts" Example
"pageCampaigns" Example
"compare" Example
"tagCloud" Example
Custom Topic's
You can also fire a event which will then only dispatch to a list of custom set Topic's.
Therefore add a function which returns an array of topics to the event in the topics
property.
Now you can register/subscribe a callback to the ResultDispatcher with that topic and get the result for that event type.
Web Components Utils
Motivation
You might want a little bit more controll of the dataflow for different reasons:
- own proxy
- analyzing and metrics
- security
- request/response modification.
For that purpose we build this library to support you when you roll your own proxy or want to route
the traffic through your current Java based soulution.
We have split this functions into 2 librarys:
java-proxy-utils
Check it out on GitHub
With this library you can easily hook into a Servlet based java proxy and prepare a request for the
FACT-Finder instance.
The Web Components work with a special CORS handler for security and authentication. Therefore you
need to route the HTTP OPTIONS request from the shop to FACT-Finder.
The proxy-utils allows you with just a few lines of code to handle that usecase.
Initialize the FACTFinderSettings
with your FACT-Finder account and password and the
url
of your FACT-Finder setup. All the necessary authentication information will be injected into the
request.
If you know what you are doing, you can also build a request from scratch.
We added a few Helper methods for convenient use cases, for example the extraction of the original
request headers:
Or a detection of the requested Service from the original request. (You maybe need the Service for
parsing into the right Object Model)
You can also work with the data response and modify it. Therefore you can use the
Java FACT-Finder Domain Model
Java FACT-Finder Domain Model
Check it out on GitHub
This little library allows you to parse a response from FACT-Finder and provides you with a Object
Model you
can work with. For example filter some results or add records.
When you are done, you just need to parse the Object Model back to a JSON format and send it with
the
original response.
We use Gson for the parsing with minimal pre settings.
When you need to, you can initialize the Gson parser with your custom settings.
You dont need to use the parser we provide. If you want you can use any other JSON parsing library.
All
JSON Schema files are located within the project.